镜像自地址
https://github.com/wikimedia/mediawiki-extensions-RevisionSlider
已同步 2024-05-25 06:12:56 +08:00
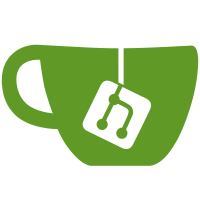
This code was added in 2016 via Iadf7793. The two CSS classes .mw-revslider-older-diff-column and .mw-revslider-newer-diff-column became unused only one month later via I317a2fc. Since then all this is apparently dead code. There are no "top borders" on these columns any more. Lines still appear in the same position, but as "border-bottom" on other elements. It really looks like we just forgot to remove this. Change-Id: I627628fa44da96ca1f1301d3a879919e4a021e5b
214 行
5.9 KiB
JavaScript
214 行
5.9 KiB
JavaScript
/**
|
|
* Module handling diff page reloading and the RevisionSlider browser history
|
|
*
|
|
* @class Diffpage
|
|
* @constructor
|
|
*/
|
|
function DiffPage() {
|
|
this.lastRequest = null;
|
|
}
|
|
|
|
$.extend( DiffPage.prototype, {
|
|
/**
|
|
* Refreshes the diff view with two given revision IDs
|
|
*
|
|
* @param {number} diff
|
|
* @param {number} oldid
|
|
* @param {SliderView} sliderView
|
|
* @param {number} [retryAttempt=0]
|
|
*/
|
|
refresh: function ( diff, oldid, sliderView, retryAttempt ) {
|
|
const self = this,
|
|
retryLimit = 2,
|
|
data = {
|
|
diff: diff,
|
|
oldid: oldid
|
|
},
|
|
params = this.getExtraDiffPageParams();
|
|
|
|
retryAttempt = retryAttempt || 0;
|
|
|
|
if ( Object.keys( params ).length > 0 ) {
|
|
$.extend( data, params );
|
|
}
|
|
|
|
if ( this.lastRequest ) {
|
|
this.lastRequest.abort();
|
|
}
|
|
|
|
$( 'table.diff[data-mw="interface"]' ).addClass( 'mw-revslider-diff-loading' );
|
|
|
|
this.lastRequest = $.ajax( {
|
|
url: mw.util.wikiScript( 'index' ),
|
|
data: data,
|
|
tryCount: 0
|
|
} );
|
|
// Don't chain, so lastRequest is a jQuery.jqXHR object
|
|
this.lastRequest.then( function ( data2 ) {
|
|
const $data = $( data2 ),
|
|
$container = $( '.mw-revslider-container' ),
|
|
scrollLeft = $container.find( '.mw-revslider-revisions-container' ).scrollLeft();
|
|
|
|
// Add our current rendered slider into the newly loaded container
|
|
$data.find( '.mw-revslider-container' ).replaceWith( $container );
|
|
const $contentText = $data.find( '#mw-content-text' );
|
|
|
|
// Replace elements on the page with the newly loaded elements, from top to bottom
|
|
$( '#mw-content-text' ).replaceWith( $contentText );
|
|
$( '.printfooter' ).replaceWith( $data.find( '.printfooter' ) );
|
|
$( '#catlinks' ).replaceWith( $data.find( '#catlinks' ) );
|
|
$( '#mw-panel' ).replaceWith( $data.find( '#mw-panel' ) );
|
|
// Update edit link
|
|
$( '#ca-edit a, #ca-ve-edit a' ).each( function () {
|
|
const uri = new mw.Uri( $( this ).attr( 'href' ) );
|
|
uri.query.oldid = diff;
|
|
$( this ).attr( 'href', uri.toString() );
|
|
} );
|
|
// Update wgRevisionId (T161257), and wgDiffOldId/NewId
|
|
mw.config.set( {
|
|
wgRevisionId: diff,
|
|
wgDiffOldId: oldid,
|
|
wgDiffNewId: diff
|
|
} );
|
|
|
|
$( '.mw-revslider-revisions-container' ).scrollLeft( scrollLeft );
|
|
|
|
self.addHandlersToCoreLinks( sliderView );
|
|
|
|
// Re-trigger existing, stable core hooks under the same circumstances as in core
|
|
mw.hook( 'wikipage.content' ).fire( $contentText );
|
|
mw.hook( 'wikipage.diff' ).fire( $( document.body ).find( 'table.diff' ) );
|
|
}, function ( xhr ) {
|
|
$( 'table.diff[data-mw="interface"]' ).removeClass( 'mw-revslider-diff-loading' );
|
|
if ( xhr.statusText !== 'abort' ) {
|
|
this.tryCount++;
|
|
mw.track( 'counter.MediaWiki.RevisionSlider.error.refresh' );
|
|
if ( retryAttempt <= retryLimit ) {
|
|
self.refresh( diff, oldid, sliderView, retryAttempt + 1 );
|
|
}
|
|
// TODO notify the user that we failed to update the diff?
|
|
// This could also attempt to reload the page with the correct diff loaded without ajax?
|
|
}
|
|
} );
|
|
},
|
|
|
|
/**
|
|
* Replaces the current state in the history stack
|
|
*
|
|
* @param {number} diff
|
|
* @param {number} oldid
|
|
* @param {SliderView} sliderView
|
|
*/
|
|
replaceState: function ( diff, oldid, sliderView ) {
|
|
history.replaceState(
|
|
this.getStateObject( diff, oldid, sliderView ),
|
|
$( document ).find( 'title' ).text(),
|
|
this.getStateUrl( diff, oldid )
|
|
);
|
|
},
|
|
|
|
/**
|
|
* Pushes the current state onto the history stack
|
|
*
|
|
* @param {number} diff
|
|
* @param {number} oldid
|
|
* @param {SliderView} sliderView
|
|
*/
|
|
pushState: function ( diff, oldid, sliderView ) {
|
|
history.pushState(
|
|
this.getStateObject( diff, oldid, sliderView ),
|
|
$( document ).find( 'title' ).text(),
|
|
this.getStateUrl( diff, oldid )
|
|
);
|
|
},
|
|
|
|
/**
|
|
* Gets a state object to be used with history.replaceState and history.pushState
|
|
*
|
|
* @private
|
|
* @param {number} diff
|
|
* @param {number} oldid
|
|
* @param {SliderView} sliderView
|
|
* @return {Object}
|
|
*/
|
|
getStateObject: function ( diff, oldid, sliderView ) {
|
|
return {
|
|
diff: diff,
|
|
oldid: oldid,
|
|
pointerOlderPos: sliderView.pointerOlder.getPosition(),
|
|
pointerNewerPos: sliderView.pointerNewer.getPosition(),
|
|
sliderPos: sliderView.slider.getOldestVisibleRevisionIndex()
|
|
};
|
|
},
|
|
|
|
/**
|
|
* Gets a URL to be used with history.replaceState and history.pushState
|
|
*
|
|
* @private
|
|
* @param {number} diff
|
|
* @param {number} oldid
|
|
* @return {string}
|
|
*/
|
|
getStateUrl: function ( diff, oldid ) {
|
|
let url = mw.util.wikiScript( 'index' ) + '?diff=' + diff + '&oldid=' + oldid;
|
|
const params = this.getExtraDiffPageParams();
|
|
for ( const key in params ) {
|
|
url += '&' + key + '=' + params[ key ];
|
|
}
|
|
return url;
|
|
},
|
|
|
|
/**
|
|
* Returns an object containing all possible parameters that should be included in diff URLs
|
|
* when selected revisions change, e.g. uselang
|
|
*
|
|
* @private
|
|
* @return {Object}
|
|
*/
|
|
getExtraDiffPageParams: function () {
|
|
const params = {},
|
|
paramArray = location.search.slice( 1 ).split( '&' ).filter( function ( elem ) {
|
|
return elem.indexOf( '=' ) > 0 && elem.match( /^(diff|oldid)=/ ) === null;
|
|
} );
|
|
paramArray.forEach( function ( elem ) {
|
|
const pair = elem.split( '=', 2 );
|
|
params[ pair[ 0 ] ] = pair[ 1 ];
|
|
} );
|
|
return params;
|
|
},
|
|
|
|
/**
|
|
* @param {SliderView} sliderView
|
|
*/
|
|
initOnPopState: function ( sliderView ) {
|
|
window.addEventListener( 'popstate', function ( event ) {
|
|
if ( event.state === null ) {
|
|
return;
|
|
}
|
|
mw.track( 'counter.MediaWiki.RevisionSlider.event.historyChange' );
|
|
sliderView.slider.setFirstVisibleRevisionIndex( event.state.sliderPos );
|
|
sliderView.updatePointersAndDiffView(
|
|
event.state.pointerNewerPos,
|
|
event.state.pointerOlderPos,
|
|
false
|
|
);
|
|
} );
|
|
},
|
|
|
|
/**
|
|
* @param {SliderView} sliderView
|
|
*/
|
|
addHandlersToCoreLinks: function ( sliderView ) {
|
|
$( '#differences-nextlink' ).on( 'click', function () {
|
|
sliderView.showNextDiff();
|
|
return false;
|
|
} );
|
|
$( '#differences-prevlink' ).on( 'click', function () {
|
|
sliderView.showPrevDiff();
|
|
return false;
|
|
} );
|
|
}
|
|
} );
|
|
|
|
module.exports = DiffPage;
|