镜像自地址
https://github.com/wikimedia/mediawiki-extensions-RevisionSlider
已同步 2024-05-25 06:12:56 +08:00
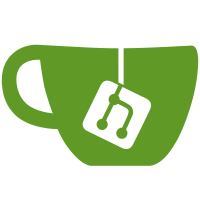
I have seen this getting fed with fractional numbers like 38.9994375. This apparently happens when specific browser zooms are used, e.g. 110% in Google Chrome. There is a lot of code that expects this to be an integer number and stops working entirely when it isn't. I'm also updating two related comparisons to not be so extremely specific any more. This probably doesn't make a difference any more with the fix above, but can't hurt. This patch doesn't solve T352169, but is one more puzzle piece on the way to solve it. Bug: T352169 Change-Id: Ied0b9748beec941e901ca4ecba428c16967ca510
140 行
3.3 KiB
JavaScript
140 行
3.3 KiB
JavaScript
const SliderView = require( './ext.RevisionSlider.SliderView.js' );
|
|
|
|
/**
|
|
* Module handling the slider logic of the RevisionSlider
|
|
*
|
|
* @class Slider
|
|
* @param {RevisionList} revisions
|
|
* @constructor
|
|
*/
|
|
function Slider( revisions ) {
|
|
this.revisions = revisions;
|
|
this.view = new SliderView( this );
|
|
}
|
|
|
|
$.extend( Slider.prototype, {
|
|
/**
|
|
* @type {RevisionList}
|
|
*/
|
|
revisions: null,
|
|
|
|
/**
|
|
* @type {number}
|
|
*/
|
|
oldestVisibleRevisionIndex: 0,
|
|
|
|
/**
|
|
* @type {number}
|
|
*/
|
|
revisionsPerWindow: 0,
|
|
|
|
/**
|
|
* @type {SliderView}
|
|
*/
|
|
view: null,
|
|
|
|
/**
|
|
* @return {RevisionList}
|
|
*/
|
|
getRevisionList: function () {
|
|
return this.revisions;
|
|
},
|
|
|
|
/**
|
|
* @return {SliderView}
|
|
*/
|
|
getView: function () {
|
|
return this.view;
|
|
},
|
|
|
|
/**
|
|
* Sets the number of revisions that are visible at once (depending on browser window size)
|
|
*
|
|
* @param {number} n
|
|
*/
|
|
setRevisionsPerWindow: function ( n ) {
|
|
this.revisionsPerWindow = Math.round( n );
|
|
},
|
|
|
|
/**
|
|
* @return {number}
|
|
*/
|
|
getRevisionsPerWindow: function () {
|
|
return this.revisionsPerWindow;
|
|
},
|
|
|
|
/**
|
|
* Returns the index of the oldest revision that is visible in the current window
|
|
*
|
|
* @return {number}
|
|
*/
|
|
getOldestVisibleRevisionIndex: function () {
|
|
return this.oldestVisibleRevisionIndex;
|
|
},
|
|
|
|
/**
|
|
* Returns the index of the newest revision that is visible in the current window
|
|
*
|
|
* @return {number}
|
|
*/
|
|
getNewestVisibleRevisionIndex: function () {
|
|
return this.oldestVisibleRevisionIndex + this.revisionsPerWindow - 1;
|
|
},
|
|
|
|
/**
|
|
* @return {boolean}
|
|
*/
|
|
isAtStart: function () {
|
|
return this.getOldestVisibleRevisionIndex() <= 0 ||
|
|
this.revisions.getLength() <= this.revisionsPerWindow;
|
|
},
|
|
|
|
/**
|
|
* @return {boolean}
|
|
*/
|
|
isAtEnd: function () {
|
|
return this.getNewestVisibleRevisionIndex() >= this.revisions.getLength() - 1 ||
|
|
this.revisions.getLength() <= this.revisionsPerWindow;
|
|
},
|
|
|
|
/**
|
|
* Sets the index of the first revision that is visible in the current window
|
|
*
|
|
* @param {number} value
|
|
*/
|
|
setFirstVisibleRevisionIndex: function ( value ) {
|
|
const highestPossibleFirstRev = this.revisions.getLength() - this.revisionsPerWindow;
|
|
value = Math.min( Math.max( 0, value ), highestPossibleFirstRev );
|
|
this.oldestVisibleRevisionIndex = value;
|
|
},
|
|
|
|
/**
|
|
* Sets the new oldestVisibleRevisionIndex after sliding in a direction
|
|
*
|
|
* @param {number} direction - Either -1, 0 or 1
|
|
*/
|
|
slide: function ( direction ) {
|
|
const value = this.oldestVisibleRevisionIndex + direction * this.revisionsPerWindow;
|
|
this.setFirstVisibleRevisionIndex( value );
|
|
}
|
|
} );
|
|
|
|
module.exports = {
|
|
Api: require( './ext.RevisionSlider.Api.js' ),
|
|
DiffPage: require( './ext.RevisionSlider.DiffPage.js' ),
|
|
HelpDialog: require( './ext.RevisionSlider.HelpDialog.js' ),
|
|
makeRevisions: require( './ext.RevisionSlider.RevisionList.js' ).makeRevisions,
|
|
Revision: require( './ext.RevisionSlider.Revision.js' ).Revision,
|
|
RevisionList: require( './ext.RevisionSlider.RevisionList.js' ).RevisionList,
|
|
RevisionListView: require( './ext.RevisionSlider.RevisionListView.js' ),
|
|
setUserOffset: require( './ext.RevisionSlider.Revision.js' ).setUserOffset,
|
|
Slider: Slider,
|
|
SliderView: SliderView,
|
|
utils: require( './ext.RevisionSlider.util.js' ),
|
|
|
|
private: {
|
|
Pointer: require( './ext.RevisionSlider.Pointer.js' ),
|
|
PointerView: require( './ext.RevisionSlider.PointerView.js' )
|
|
}
|
|
};
|