镜像自地址
https://github.com/wikimedia/mediawiki-extensions-Math.git
已同步 2024-06-02 15:50:20 +08:00
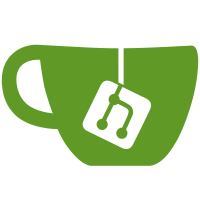
Adding action=purge to the url did not bypass cache in native mode, because the check request was performed before the purge information was passed. * Move purge property to the base class * Pass purge option via the checker constructor * Add cache checking for mathoid checker * Adjust method signatures accordingly Change-Id: I6f545060ae72dac8b12fb0f85662c4048059b2e9
75 行
2.1 KiB
PHP
75 行
2.1 KiB
PHP
<?php
|
|
/**
|
|
* MediaWiki math extension
|
|
*
|
|
* @copyright 2002-2023 various MediaWiki contributors
|
|
* @license GPL-2.0-or-later
|
|
*/
|
|
|
|
namespace MediaWiki\Extension\Math;
|
|
|
|
use MediaWiki\Extension\Math\InputCheck\LocalChecker;
|
|
use MediaWiki\Extension\Math\WikiTexVC\MMLnodes\MMLmath;
|
|
use MediaWiki\MediaWikiServices;
|
|
use MediaWiki\SpecialPage\SpecialPage;
|
|
use MediaWiki\Title\Title;
|
|
use StatusValue;
|
|
|
|
/**
|
|
* Converts LaTeX to MathML using PHP
|
|
*/
|
|
class MathNativeMML extends MathMathML {
|
|
private LocalChecker $checker;
|
|
|
|
public function __construct( $tex = '', $params = [], $cache = null ) {
|
|
parent::__construct( $tex, $params, $cache );
|
|
$this->setMode( MathConfig::MODE_NATIVE_MML );
|
|
}
|
|
|
|
protected function doRender(): StatusValue {
|
|
$checker = $this->getChecker();
|
|
$checker->setContext( $this );
|
|
$checker->setHookContainer( MediaWikiServices::getInstance()->getHookContainer() );
|
|
$presentation = $checker->getPresentationMathMLFragment();
|
|
$config = MediaWikiServices::getInstance()->getMainConfig();
|
|
$attributes = [ 'class' => 'mwe-math-element' ];
|
|
if ( $this->getID() !== '' ) {
|
|
$attributes['id'] = $this->getID();
|
|
}
|
|
if ( $config->get( 'MathEnableFormulaLinks' ) &&
|
|
isset( $this->params['qid'] ) &&
|
|
preg_match( '/Q\d+/', $this->params['qid'] ) ) {
|
|
$titleObj = Title::newFromLinkTarget( SpecialPage::getTitleValueFor( 'MathWikibase' ) );
|
|
$attributes['href'] = $titleObj->getLocalURL( [ 'qid' => $this->params['qid'] ] );
|
|
}
|
|
if ( $this->getMathStyle() == 'display' ) {
|
|
$attributes['display'] = 'block';
|
|
}
|
|
$root = new MMLmath( "", $attributes );
|
|
|
|
$this->setMathml( $root->encapsulateRaw( $presentation ?? '' ) );
|
|
return StatusValue::newGood();
|
|
}
|
|
|
|
protected function getChecker(): LocalChecker {
|
|
$this->checker ??= Math::getCheckerFactory()
|
|
->newLocalChecker( $this->tex, $this->getInputType(), $this->isPurge() );
|
|
return $this->checker;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getHtmlOutput(): string {
|
|
return $this->getMathml();
|
|
}
|
|
|
|
public function readFromCache(): bool {
|
|
return false;
|
|
}
|
|
|
|
public function writeCache() {
|
|
return true;
|
|
}
|
|
}
|